pytest-Painless Testing with Pytest Simplify Your Testing Process
pytest is a popular testing framework for Python developers which is known for its simplicity and ease of use. As the name suggests, pytest makes testing painless by simplifying the testing process. This article will explore the features of pytest and how it can be used to improve the testing process.
Installation
The first step to using pytest is to install it. Simply run the following command in your terminal:
pip install pytest
Writing Tests
Once pytest is installed, it's time to start writing tests. In pytest, tests are simply Python functions with names beginning with "test_". For example:
def test_addition():
assert 1 + 1 == 2
This test function asserts that 1 + 1 should equal 2. When running this test with pytest, the output should be "1 passed".
Fixtures
Another great feature of pytest is fixtures. Fixtures are objects that are created before each test function and destroyed afterwards. They can be used to set up test data or provide common functionality, such as a database connection.
Here's an example of a fixture that provides a database connection:
import pytest
import psycopg2
@pytest.fixture()
def db_connection():
conn = psycopg2.connect(database="testdb", user="testuser", password="testpass", host="localhost", port="5432")
yield conn
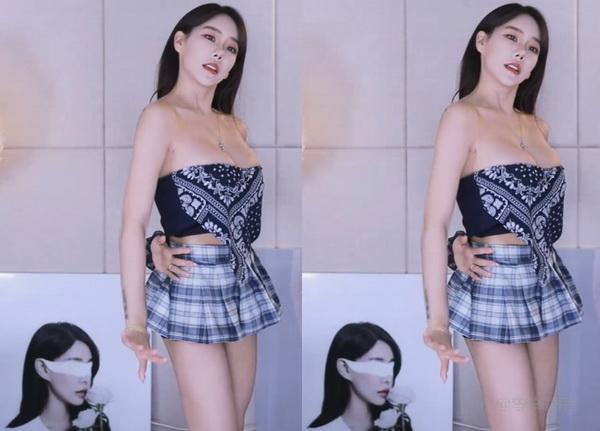
conn.close()
This fixture uses psycopg2 to create a connection to a PostgreSQL database. When the fixture is used in a test function, the connection is created and passed to the function as an argument. After the test function is completed, the fixture closes the connection.
Parameterized Tests
Parameterized tests are another useful feature of pytest. They allow you to run the same test code with multiple sets of data. This is useful for testing functions that should produce the same output for different inputs.
Here's an example of a parameterized test:
import pytest
@pytest.mark.parametrize("input, expected_output", [
("hello", "olleh"),
("world", "dlrow"),
("pytest", "tseyp")
])
def test_reverse_string(input, expected_output):
assert input[::-1] == expected_output
This test function asserts that a function that reverses a string produces the correct output for different inputs. The @pytest.mark.parametrize decorator specifies the sets of input and expected output for the test function. When the test is run with pytest, it will run the test function three times, once for each set of inputs and expected outputs.
Assertions
Assertions are a key part of testing, and pytest provides a wide range of assertions that can be used to check the output of functions. Here are a few commonly used assertions:
assert x == y
: Checks that x is equal to y
assert x != y
: Checks that x is not equal to y
assert x in y
: Checks that x is in y
assert x not in y
: Checks that x is not in y
Mocking
When writing tests, it's often necessary to mock external dependencies, such as APIs or databases. pytest provides a mocking library called pytest-mock, which can be used to easily mock external dependencies. Here's an example:
import pytest
import requests
def get_weather(city):
response = requests.get(f"https://api.openweathermap.org/data/2.5/weather?q={city}&appid=xyz")
return response.json()["weather"][0]["main"]
def test_get_weather(mocker):
mocker.patch("requests.get")
requests.get.return_value.json.return_value = {"weather": [{"main": "sunny"}]}
assert get_weather("London") == "sunny"
This test function checks that the get_weather function correctly retrieves the weather for a given city using the external OpenWeatherAPI. The mocker fixture provided by pytest-mock is used to patch the requests.get function, so that it returns a JSON response containing the weather data for the given city. This allows the test to run without actually making a network request to the API.
Conclusion
Pytest is a simple yet powerful testing framework that can greatly improve the testing process for Python developers. By providing tools such as fixtures, parameterized tests, and assertions, pytest simplifies the writing and execution of tests. Additionally, the pytest-mock library makes it easy to mock external dependencies for testing. By using pytest, developers can ensure that their code is thoroughly tested and reliable.